List Comprehension in Python
Last Updated: 22nd June, 2023
Overview:
List comprehension is a powerful and concise way to create a new list đđ. This lesson post covers its syntax đ, usage đ», and best practices.
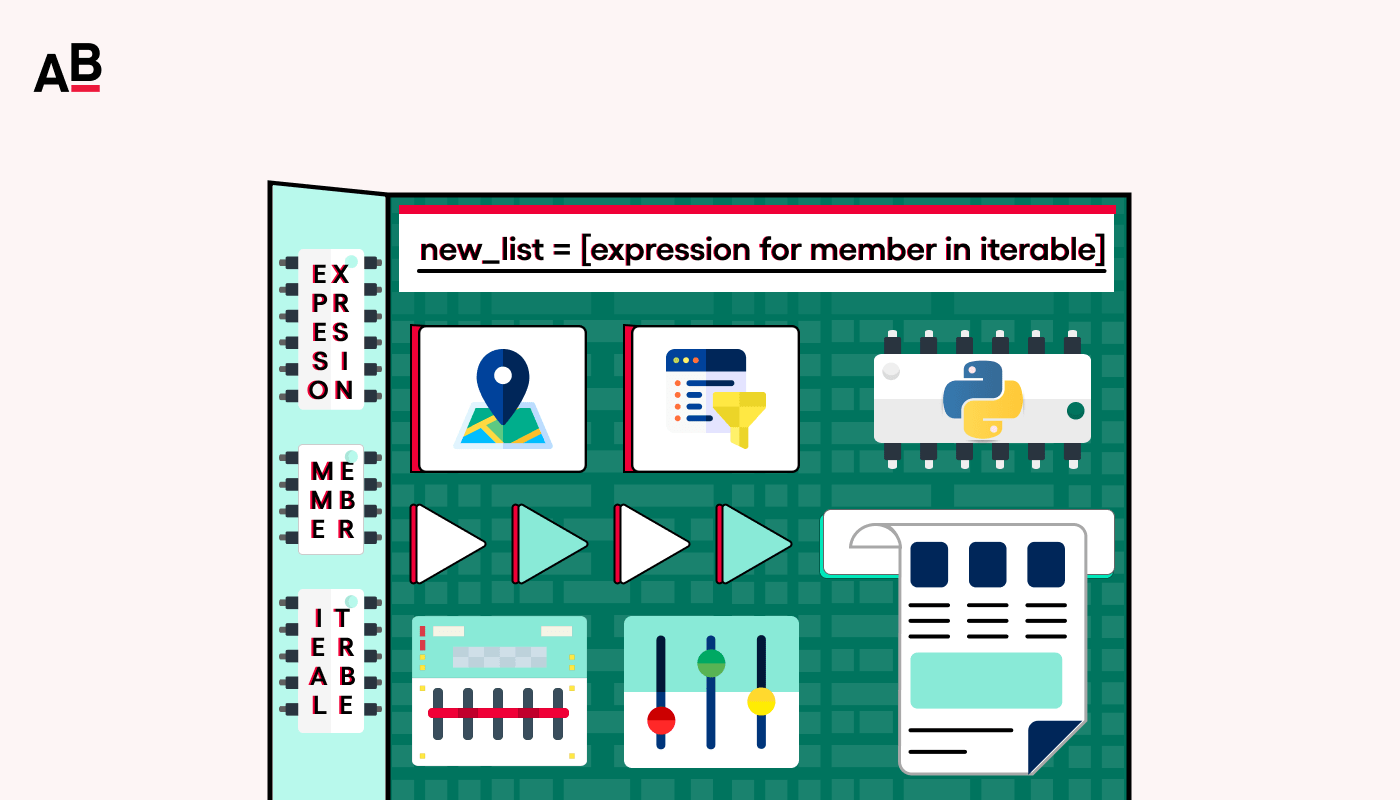
What is List Comprehension?
List comprehension could be a quick and exquisite way to form a new list in Python by iterating over an existing iterable, such as a list, tuple, string, or range, and applying a few changes or filtering to its elements. It permits you to write code more expressively and can spare time and exertion.
Syntax
List comprehension has a simple and elegant syntax that consists of three parts:
- new_list: the new list you want to create.
- Expression: an expression that defines the value of each element in the new list.
- Variable: a variable that takes each value in the iterable object.
- Iterable: an iterable object, such as a list, a tuple, or a range, that provides values for the variable.
- Condition (optional): a conditional statement that filters the elements in the iterable object.
The for loop and the if statement are the two fundamental building blocks of list comprehension. The for loop iterates over the iterable object, and the if statement filters the elements based on a condition.
Examples
Let's see some examples of list comprehension in action.
Creating a new list of squared values
In this example, we're creating a new list called squared_numbers that contains the squared values of the elements in the numbers list.
Filtering elements in a list
In this example, we're creating a new list called even_numbers that contains only the even numbers from the numbers list.
Nested list comprehension
In this example, we're creating a new list called flattened_matrix that contains all the elements in the matrix list. We're using a nested list comprehension with two for loops to achieve this.
Best Practices
Here are some best practices to follow when using list comprehension in your code:
- Please keep it simple:Â List comprehension is meant to simplify your code, so avoid using complex expressions or nesting too many for loops.
- Use meaningful variable names:Â Use variable names that convey the purpose of the variable so it's easier to understand what the list comprehension is doing.
- Use brackets to increase readability:Â Use brackets to enclose the list comprehension so it's easier to distinguish from regular code.
Conclusion
List comprehension is a powerful and elegant feature of Python that allows you to create new lists easily. It can help you write more concise and readable code and save you time and effort. Following best practices and using list comprehension effectively can make your Python code more efficient and expressive.
Key Takeaways
- List comprehension may concisely make a new list in Python by emphasizing an existing iterable, such as a list, tuple, string, or range, and applying a few changes or sifting to its components.
- Its syntax comprises three parts: the new list, an expression defining the value of each element within the unused list, a variable that takes each esteem within the iterable, the iterable question, and a discretionary condition to channel components.
- List comprehension permits you to compose code more expressively, and it can spare you a part of time and effort.
- Best practices when utilizing list comprehension include keeping it basic, using important variable names, and utilizing brackets to extend lucidness.
Quiz
- What is list comprehension in Python?
- A way to print out elements of a list
- A way to create a new list by iterating over an existing iterable and applying some transformation or filtering to its elements.
- A way to delete elements from a list
- A way to sort elements in a list
Answer: b. A way to create a new list by iterating over an existing iterable and applying some transformation or filtering to its elements.
- What are the building blocks of list comprehension?
- While loop and for loop
- For loop and if statement
- If statement and elif statement
- Try statement and except statement
Answer: b. For loop and if statement
- What is the purpose of using meaningful variable names in list comprehension?
- To make the code more complex
- To make the code harder to read
- To make the code easier to understand
- To make the code run faster
Answer: c. To make the code easier to understand
- What are some best practices to follow when using list comprehension in your code?
- Keep it complex and use vague variable names
- Use brackets to decrease readability
- Use meaningful variable names and keep it simple
- Use nested for loops and if statements
Answer:c. Use meaningful variable names and keep it simple